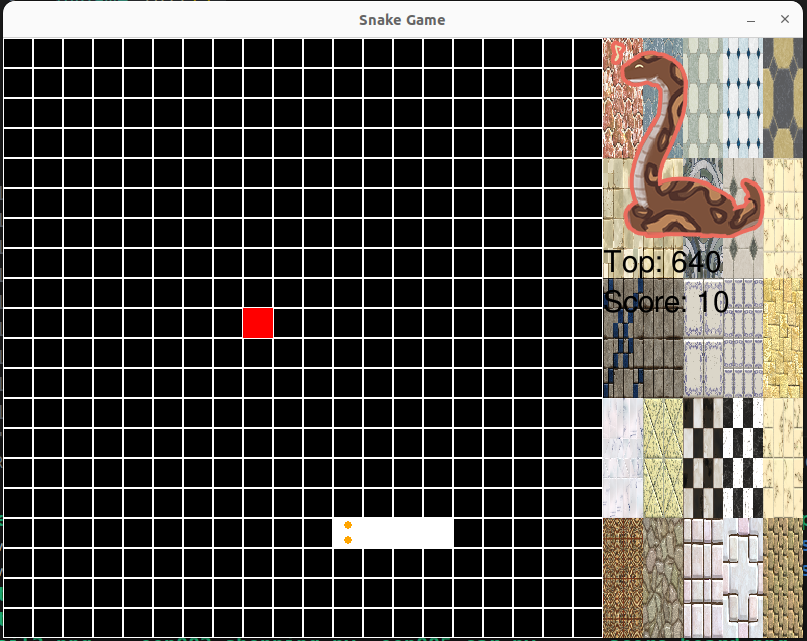
snake game(贪吃蛇)
分解snake game游戏,利用现有的知识构建完整的程序
变量
循环
贪吃蛇游戏的基本逻辑:蛇移动、碰撞处理、蛇身体增长
导入外部库
# 面向函数的编程范式,不涉及class,对象等
import pygame
import random
定义一些变量,保存游戏面板大小与颜色等
# 定义一些颜色变量
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 游戏屏幕大小
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 600
# 游戏面板行列,以及每个格子大小
BOARD_ROWS = 20
BOARD_COLS = 20
GRID_SIZE = 30
初始化pygame
# 初始化pygame,调用pygame方法之前必须初始化
pygame.init()
# 新建一个显示屏幕变量
screen = pygame.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
# 设置窗口标题栏
pygame.display.set_caption("Snake Game")
定义snake和food变量
# 定义snake和food变量
snake = [(3, 0), (2, 0), (1, 0)]
direction = "RIGHT"
food_position = (random.randint(0, BOARD_COLS - 1), random.randint(0, BOARD_ROWS - 1))
定义函数 移动snake和生成food
# move_snake函数,以及生成food的函数
def move_snake():
global snake
head = snake[0]
if direction == "RIGHT":
new_head = (head[0] + 1, head[1])
elif direction == "LEFT":
new_head = (head[0] - 1, head[1])
elif direction == "UP":
new_head = (head[0], head[1] - 1)
elif direction == "DOWN":
new_head = (head[0], head[1] + 1)
snake.insert(0, new_head)
snake.pop()
def generate_food():
global food_position
food_position = (random.randint(0, BOARD_COLS - 1), random.randint(0, BOARD_ROWS - 1))
定义函数-绘制游戏面板,snake以及food
# Define the function for drawing the board
def draw_board():
for row in range(BOARD_ROWS):
for col in range(BOARD_COLS):
x = col * GRID_SIZE
y = row * GRID_SIZE
pygame.draw.rect(screen, BLUE, [x, y, GRID_SIZE, GRID_SIZE], 1)
# Define the function for drawing the snake
def draw_snake():
for cell in snake:
x = cell[0] * GRID_SIZE
y = cell[1] * GRID_SIZE
pygame.draw.rect(screen, GREEN, [x, y, GRID_SIZE, GRID_SIZE])
# Define the function for drawing the food
def draw_food():
x = food_position[0] * GRID_SIZE
y = food_position[1] * GRID_SIZE
pygame.draw.rect(screen, WHITE, [x, y, GRID_SIZE, GRID_SIZE])
定义游戏clock,决定了游戏刷新频率
# Set the clock for the game
clock = pygame.time.Clock()
游戏退出标志,True退出游戏
# Set the game loop flag
done = False
游戏的主循环,处理event,更新游戏状态:food,snake等,重新绘制food,snake,board等
# Start the game loop
while not done:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT and direction != "LEFT":
direction = "RIGHT"
elif event.key == pygame.K_LEFT and direction != "RIGHT":
direction = "LEFT"
elif event.key == pygame.K_UP and direction != "DOWN":
direction = "UP"
elif event.key == pygame.K_DOWN and direction != "UP":
direction = "DOWN"
elif event.key == pygame.K_ESCAPE:
done = True
# Move the Snake
move_snake()
# Check if the Snake collides
# Check if the Snake collides with the Food
if snake[0] == food_position:
generate_food()
snake.append(snake[-1])
# Check if the Snake collides with the wall
if snake[0][0] < 0 or snake[0][0] >= BOARD_COLS or snake[0][31] < 0 or snake[0][32] >= BOARD_ROWS:
done = True
# Check if the Snake collides with its own body
for i in range(1, len(snake)):
if snake[0] == snake[i]:
done = True
# Clear the screen
screen.fill(BLACK)
# Draw the board, Snake, and Food
draw_board()
draw_snake()
draw_food()
# Update the screen
pygame.display.flip()
# Set the frame rate of the game
clock.tick(5)
退出游戏,关闭pygame
pygame.quit()