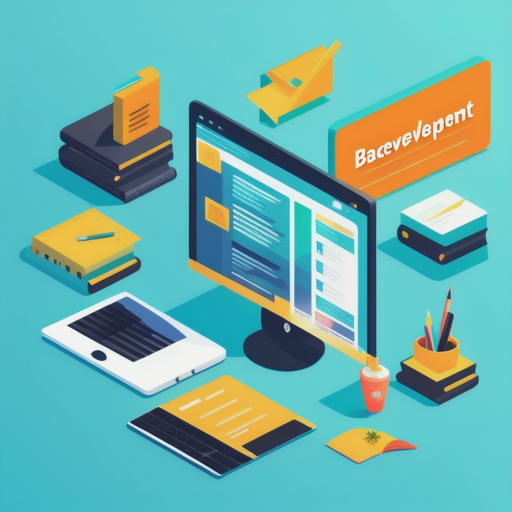
继续简单的练习,新增一个菜单项,让用户(这里并没有区分管理员或者普通读者)可以新增图书
'''
ver8.py: 新增图书 菜单项
'''
import json
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
self.is_checked_out = False
self.borrow_date = None
def display_info(self):
status = "已借出" if self.is_checked_out else "可借阅"
print(f"《{self.title}》 - 作者: {self.author} - 状态: {status}")
def check_out(self):
self.is_checked_out = True
self.borrow_date = "今天" # 在实际应用中,可以使用更复杂的日期逻辑
def check_in(self):
self.is_checked_out = False
self.borrow_date = None
class User:
def __init__(self, username, password):
self.username = username
self.password = password
self.borrowed_books = []
def borrow_book(self, book):
if not book.is_checked_out:
book.check_out()
self.borrowed_books.append(book)
print(f"{self.username}借阅了《{book.title}》。")
else:
print("抱歉,这本书已经被借出了。")
def return_book(self, book):
if book in self.borrowed_books:
book.check_in()
self.borrowed_books.remove(book)
print(f"{self.username}归还了《{book.title}》。")
else:
print("这本书没有被这位用户借阅。")
class Library:
def __init__(self, name):
self.name = name
self.books = []
self.users = []
self.current_user = None
self.borrowed_books = []
# 从文件加载图书信息
self.load_books_from_file()
# 从文件加载用户信息
self.load_users_from_file()
def load_books_from_file(self):
try:
with open(f"{self.name}_books.json", "r") as file:
books_data = json.load(file)
for book_data in books_data:
book = Book(book_data["title"], book_data["author"])
book.is_checked_out = book_data["is_checked_out"]
book.borrow_date = book_data["borrow_date"]
self.books.append(book)
except FileNotFoundError:
# 文件不存在,可能是第一次运行程序
pass
def load_users_from_file(self):
try:
with open(f"{self.name}_users.json", "r") as file:
users_data = json.load(file)
for user_data in users_data:
user = User(user_data["username"], user_data["password"])
user.borrowed_books = user_data["borrowed_books"]
self.users.append(user)
except FileNotFoundError:
# 文件不存在,可能是第一次运行程序
pass
def save_books_to_file(self):
with open(f"{self.name}_books.json", "w") as file:
books_data = []
for book in self.books:
book_data = {
"title": book.title,
"author": book.author,
"is_checked_out": book.is_checked_out,
"borrow_date": book.borrow_date,
}
books_data.append(book_data)
json.dump(books_data, file, indent=2)
def save_users_to_file(self):
with open(f"{self.name}_users.json", "w") as file:
users_data = []
for user in self.users:
user_data = {
"username": user.username,
"password": user.password,
"borrowed_books": [book.title for book in user.borrowed_books],
}
users_data.append(user_data)
json.dump(users_data, file, indent=2)
def list_books(self):
print(f"{self.name}图书馆目录:")
for book in self.books:
book.display_info()
def add_new_book(self):
title = input("请输入新书的标题: ")
author = input("请输入新书的作者: ")
new_book = Book(title, author)
self.books.append(new_book)
print(f"《{title}》已成功添加到图书馆。")
self.save_books_to_file() # 保存图书信息到文件
def search_book_by_name(self, book_name):
for book in self.books:
if book.title == book_name:
return book
return None
def check_book_status(self, book_name):
book = self.search_book_by_name(book_name)
if book:
if book.is_checked_out:
print(f"《{book_name}》已经被借出。")
else:
print(f"《{book_name}》可供借阅。")
else:
print(f"在{self.name}图书馆中找不到《{book_name}》。")
def user_login(self, username, password):
for user in self.users:
if user.username == username and user.password == password:
self.current_user = user
return user
return None
def user_registration(self, username, password):
new_user = User(username, password)
self.users.append(new_user)
print(f"{self.name}图书馆用户 '{username}' 注册成功。")
self.save_users_to_file() # 保存用户信息到文件
def user_logout(self):
print(f"再见,{self.current_user.username}!")
self.current_user = None
def borrow_book(self, book):
if not book.is_checked_out:
book.check_out()
self.current_user.borrowed_books.append(book)
self.borrowed_books.append({"user": self.current_user.username, "book": book.title})
print(f"{self.current_user.username}借阅了《{book.title}》。")
self.save_books_to_file() # 保存图书信息到文件
self.save_users_to_file() # 保存用户信息到文件
else:
print("抱歉,这本书已经被借出了。")
def return_book(self, book):
if book in self.current_user.borrowed_books:
book.check_in()
self.current_user.borrowed_books.remove(book)
self.borrowed_books = [record for record in self.borrowed_books if record["book"] != book.title]
print(f"{self.current_user.username}归还了《{book.title}》。")
self.save_books_to_file() # 保存图书信息到文件
self.save_users_to_file() # 保存用户信息到文件
else:
print("这本书没有被这位用户借阅。")
# 创建图书馆对象
library_name = input("请输入图书馆名字: ")
library = Library(library_name)
# 打印欢迎信息
print(f"欢迎使用{library.name}图书馆!")
while True:
print("\n图书馆菜单:")
print("1. 列出图书")
print("2. 检查图书状态")
print("3. 用户登录")
print("4. 用户注册")
print("5. 新增图书")
print("6. 退出登录")
print("7. 退出程序")
choice = input("请输入您的选择 (1-7): ")
if choice == "1":
library.list_books()
elif choice == "2":
book_name = input("请输入书名: ")
library.check_book_status(book_name)
elif choice == "3":
username = input("请输入用户名: ")
password = input("请输入密码: ")
logged_in_user = library.user_login(username, password)
if logged_in_user:
print(f"欢迎,{logged_in_user.username}!")
# 用户操作(借书、还书等)
while True:
print("\n用户菜单:")
print("1. 借阅图书")
print("2. 归还图书")
print("3. 退出登录")
user_choice = input("请输入您的选择 (1-3): ")
if user_choice == "1":
book_name = input("请输入要借阅的书名: ")
book = library.search_book_by_name(book_name)
if book:
library.borrow_book(book)
else:
print("找不到这本书。")
elif user_choice == "2":
book_name = input("请输入要归还的书名: ")
book = library.search_book_by_name(book_name)
if book:
library.return_book(book)
else:
print("找不到这本书。")
elif user_choice == "3":
library.user_logout()
break
else:
print("无效的选择。请输入1到3之间的数字。")
else:
print("登录失败。用户名或密码无效。")
elif choice == "4":
username = input("请输入新的用户名: ")
password = input("请输入新的密码: ")
library.user_registration(username, password)
elif choice == "5":
library.add_new_book()
elif choice == "6":
if library.current_user:
library.user_logout()
else:
print("当前没有用户登录。")
elif choice == "7":
print(f"退出{library.name}图书馆。再见!")
break
else:
print("无效的选择。请输入1到7之间的数字。")